# Nuxt
# Creating an app
npm install --save nuxt
npm install -g create-nuxt-app
create-nuxt-app frontend
cd frontend
npm install feathers-vuex @vue/composition-api --save
npm run dev
1
2
3
4
5
6
7
8
9
10
11
2
3
4
5
6
7
8
9
10
11
dark mode toggle
<v-btn @click="$vuetify.theme.dark=!$vuetify.theme.dark">
<v-icon dark>
mdi-theme-light-dark
</v-icon>
</v-btn>
1
2
3
4
5
2
3
4
5
or install color-mode pkg here
Feathers Vuex
Layout.vue
<template>
<v-app dark>
<v-navigation-drawer
v-model="drawer"
:mini-variant="miniVariant"
:clipped="clipped"
fixed
app
>
<v-list>
<v-list-item
v-for="(item, i) in items"
:key="i"
:to="item.to"
router
exact
>
<v-list-item-action>
<v-icon>{{ item.icon }}</v-icon>
</v-list-item-action>
<v-list-item-content>
<v-list-item-title v-text="item.title" />
</v-list-item-content>
</v-list-item>
</v-list>
</v-navigation-drawer>
<v-app-bar
:clipped-left="clipped"
fixed
app
>
<v-app-bar-nav-icon @click.stop="drawer = !drawer" />
<v-btn
icon
@click.stop="miniVariant = !miniVariant"
>
<v-icon>mdi-{{ `chevron-${miniVariant ? 'right' : 'left'}` }}</v-icon>
</v-btn>
<!-- <v-btn-->
<!-- icon-->
<!-- @click.stop="clipped = !clipped"-->
<!-- >-->
<!-- <v-icon>mdi-application</v-icon>-->
<!-- </v-btn>-->
<!-- <v-btn-->
<!-- icon-->
<!-- @click.stop="fixed = !fixed"-->
<!-- >-->
<!-- <v-icon>mdi-minus</v-icon>-->
<!-- </v-btn>-->
<v-btn icon @click="$vuetify.theme.dark=!$vuetify.theme.dark">
<v-icon dark>
mdi-theme-light-dark
</v-icon>
</v-btn>
<v-spacer />
<v-toolbar-title v-text="title" />
<v-spacer />
<v-btn
icon
@click.stop="rightDrawer = !rightDrawer"
to="login"
>
<v-icon>mdi-chat</v-icon>
</v-btn>
</v-app-bar>
<v-main>
<v-container>
<Nuxt />
</v-container>
</v-main>
<v-navigation-drawer
v-model="rightDrawer"
:right="right"
temporary
fixed
>
<v-list>
<v-list-item @click.native="right = !right">
<v-list-item-action>
<v-icon light>
mdi-repeat
</v-icon>
</v-list-item-action>
<v-list-item-title>Switch drawer (click me)</v-list-item-title>
</v-list-item>
</v-list>
</v-navigation-drawer>
<v-footer
:absolute="!fixed"
app
>
<span>© {{ new Date().getFullYear() }}</span>
</v-footer>
</v-app>
</template>
<script>
export default {
name: 'DefaultLayout',
data () {
return {
clipped: true,
drawer: false,
fixed: false,
items: [
{
icon: 'mdi-home',
title: 'Welcome',
to: '/'
},
{
icon: 'mdi-apps',
title: 'Poetry',
to: '/poetry'
},
{
icon: 'mdi-chat',
title: 'Chat',
to: '/chat'
}
],
miniVariant: false,
right: true,
rightDrawer: false,
title: 'Poesias de Ara'
}
}
}
</script>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
TIP
Use "socket.io-client": "^2.3.1",
instead of "socket.io-client": "^4.4.1",
, feathers 4 doesn't support socket.io greater than 3.
# ESlint and Prettir configuration for a Nuxt App
npm install --save-dev babel-eslint eslint eslint-config-prettier eslint-loader eslint-plugin-vue eslint-plugin-prettier prettier
1
2
2
creat a .eslintrc.js
file in the root folder
export default {
env: {
browser: true,
node: true,
es2021: true,
},
extends: [
// 'standard',
"plugin:vue/essential",
"plugin:vue/recommended",
"plugin:prettier/recommended",
// 'prettier/vue',
"eslint:recommended",
],
parserOptions: {
ecmaVersion: 2018,
parser: "babel-eslint",
// parser: '@typescript-eslint/parser',
sourceType: "module",
},
plugins: [
"vue",
// '@typescript-eslint'
],
rules: {
"vue/multi-word-component-names": "warn",
"no-unused-vars": "warn",
"space-in-parens": "off",
"computed-property-spacing": "off",
},
};
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
nuxt.config.js
, add this tio the build:
build: {
transpile: [
'feathers-vuex'
],
extend(config, ctx) {
//Run ESLint on save
if (ctx.isDev && ctx.isClient) {
config.module.rules.push({
enforce: "pre",
test: /\.(js|vue|ts)$/,
loader: "eslint-loader",
exclude: /(node_modules)/
})
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
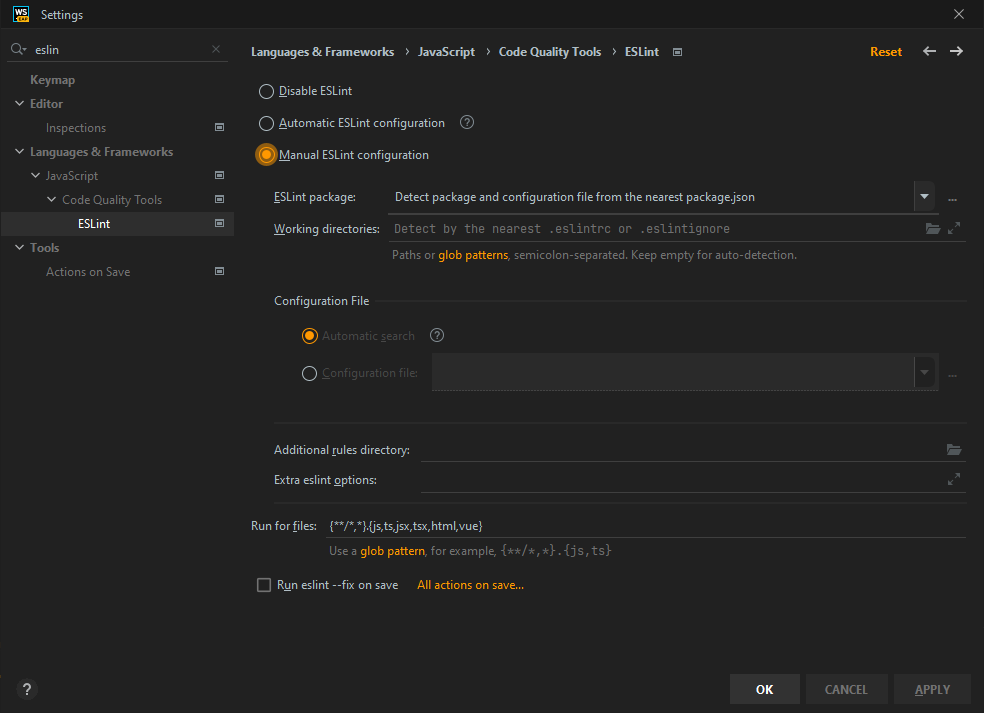