# Modern C++
# Initial definitions
# What is C++
- General Purpose programming language
- Object-oriented, imperative, generic
- Created by Bjarne Stroustrup
- Emphasis on system programming
- Low-level like C, but feature-rich
# What is Modern C++
- Encompass features of C++11
- Move semantics
- Smart pointers
- Automatic type inference
- Threading
- Lambda functions
# ISO Standard Committee
- Responsible for adding new features to C++
- Has members from all over the world
- Some are representatives of their companies (Microsoft, Google, IBM, etc)
- Published first standard in 1998, followed by a minor revision in 2003
- Major change in 2011, with lots of new features
- 2014 added a minor change, mostly enhancements
# Visual Studio Project Structure
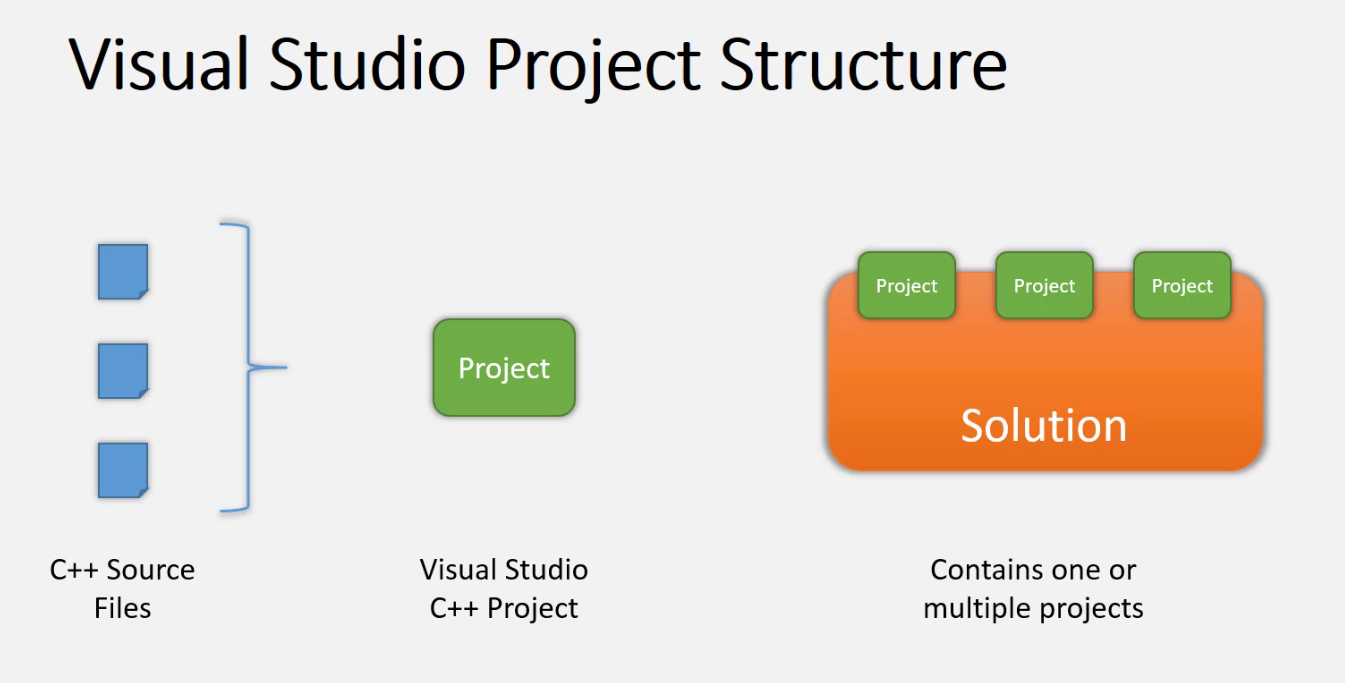
// This is a comment
/*
This is amulti
line comment
*/
#include <iostream>
using namespace std;
int main()
{
cout << "Hello World!" << "C++" << endl;
return 0;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
# Inheritance and Composition
# Object Oriented Programming
- Systems are made up of objects that represent
- Objects is an instance of a class
- Classes & Objects are related
- Collaboration between objects
- Define the behavior of the system
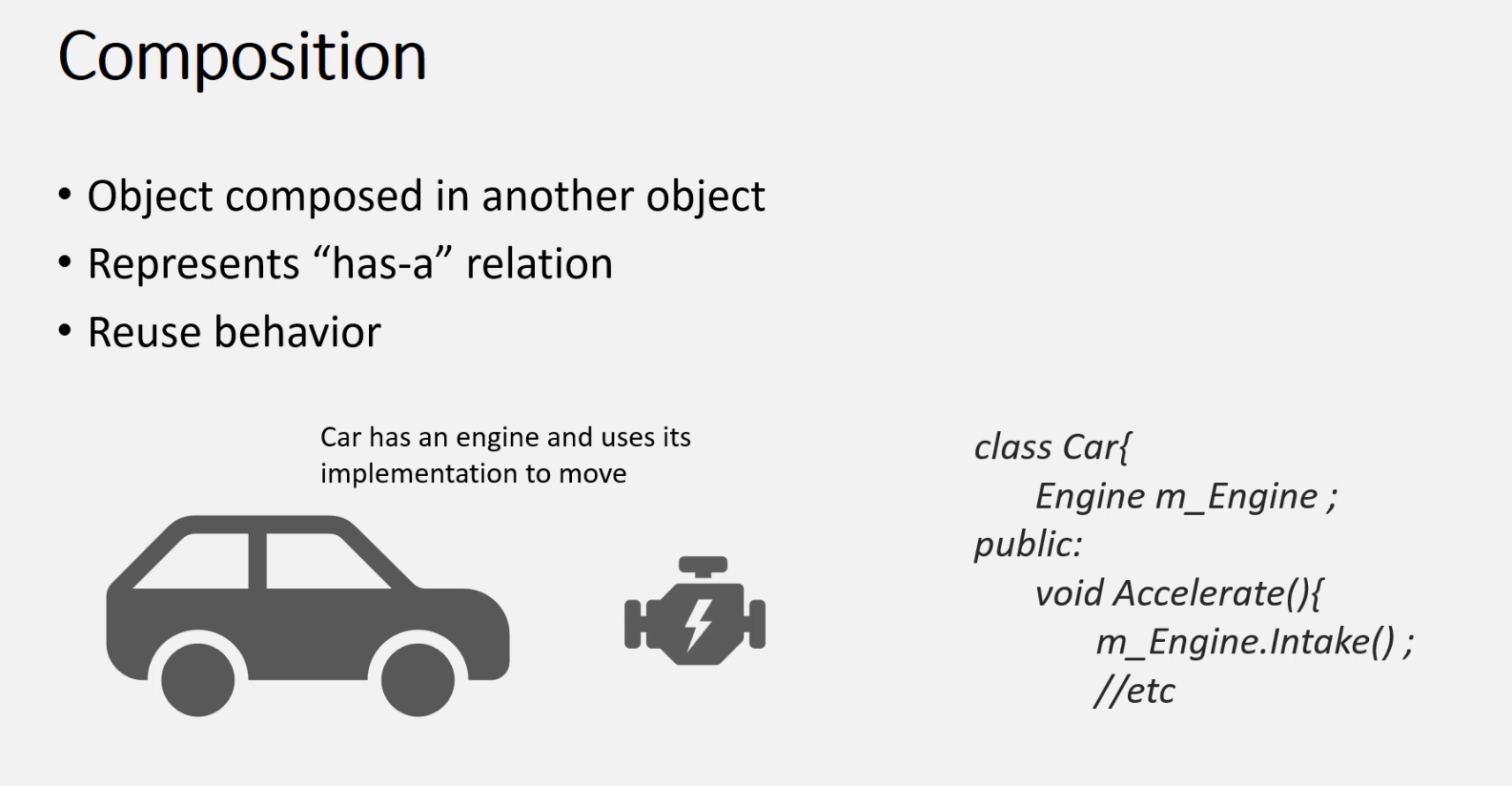
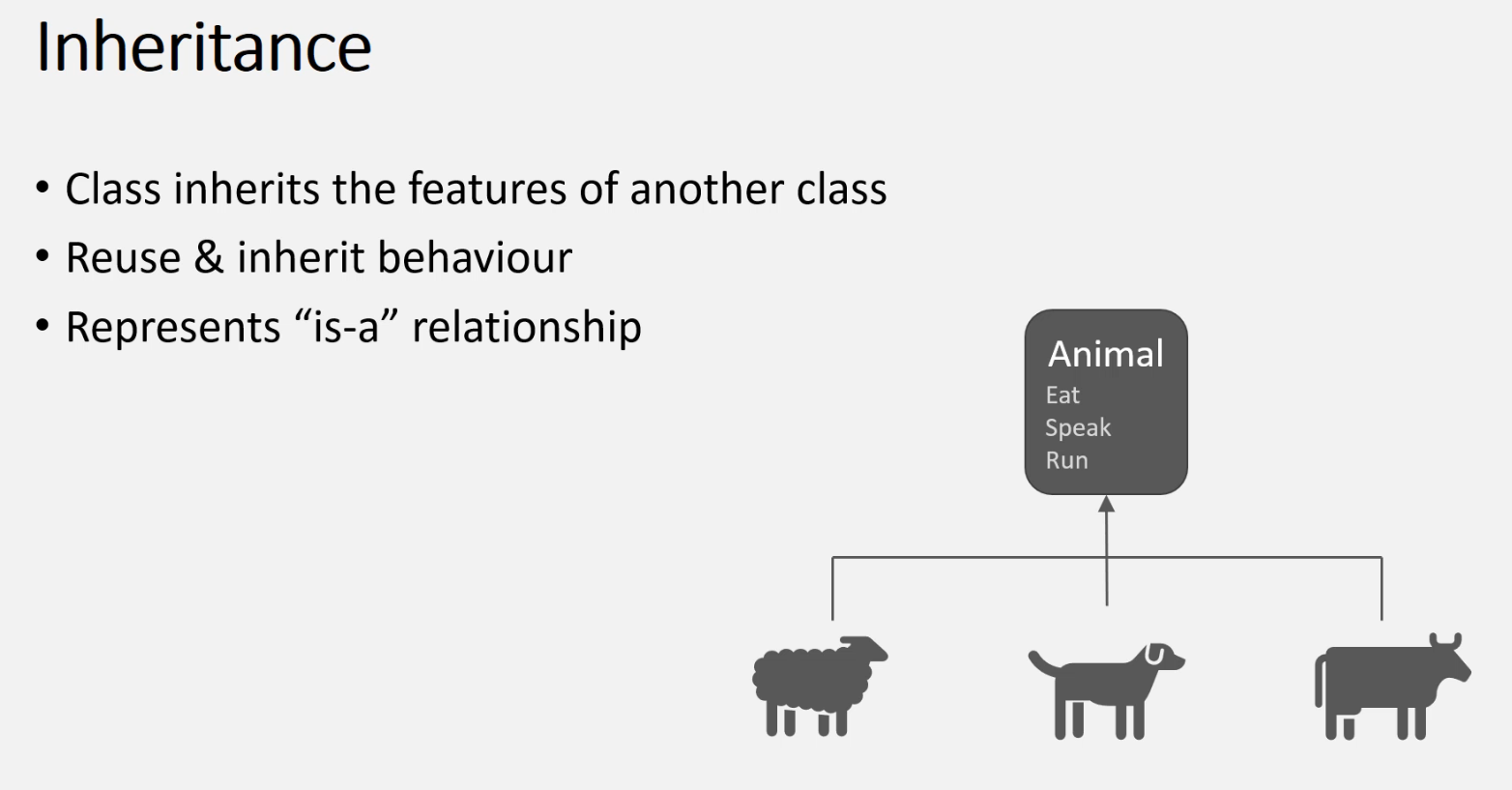
# Syntax of inheritance
class<child class> : <access modifier><base class>
the access modifier
is optional
Example:
#include <iostream>
class Animal {
public:
void Eat() {
std::cout << "Animal eating" << std::endl;
}
void Run() {
std::cout << "Animal running" << std::endl;
}
void Speak() {
std::cout << "Animal speaking" << std::endl;
}
};
class Dog : public Animal {
};
int main() {
Dog d;
d.Eat();
d.Run();
d.Speak();
return 0;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
output:
Animal eating
Animal running
Animal speaking
2
3
We can also provide particular behaviours
#include <iostream>
class Animal {
public:
void Eat() {
std::cout << "Animal eating" << std::endl;
}
void Run() {
std::cout << "Animal running" << std::endl;
}
void Speak() {
std::cout << "Animal speaking" << std::endl;
}
};
class Dog : public Animal {
public:
void Eat() {
std::cout << "Dog eating meat" << std::endl;
}
void Speak() {
std::cout << "Dog barking" << std::endl;
}
};
int main() {
Dog d;
d.Eat();
d.Run();
d.Speak();
return 0;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
output:
Dog eating meat
Animal running
Dog barking
2
3
Although we get this warning from CLion:
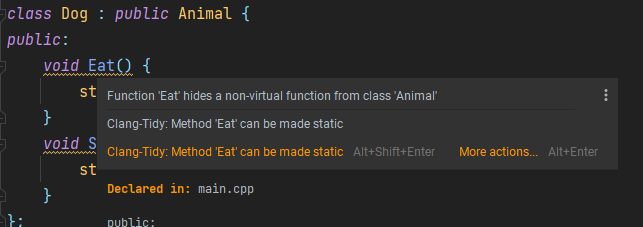
To correct this we have to make the functions static and also call direct from the class:
#include <iostream>
class Animal {
public:
static void Eat() {
std::cout << "Animal eating" << std::endl;
}
static void Run() {
std::cout << "Animal running" << std::endl;
}
static void Speak() {
std::cout << "Animal speaking" << std::endl;
}
};
class Dog : public Animal {
public:
static void Eat() {
std::cout << "Dog eating meat" << std::endl;
}
static void Speak() {
std::cout << "Dog barking" << std::endl;
}
};
int main() {
Dog::Eat();
Dog::Run();
Dog::Speak();
return 0;
}
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
TIP
Static void method is a static method which does not return anything. Static method can be called by directly by class name. void method is a method which also return nothing.
By declaring a function member as static, you make it independent of any particular object of the class. A static member function can be called even if no objects of the class exist and the static functions are accessed using only the class name and the scope resolution operator ::
.
# Inheritance and Access Modifiers
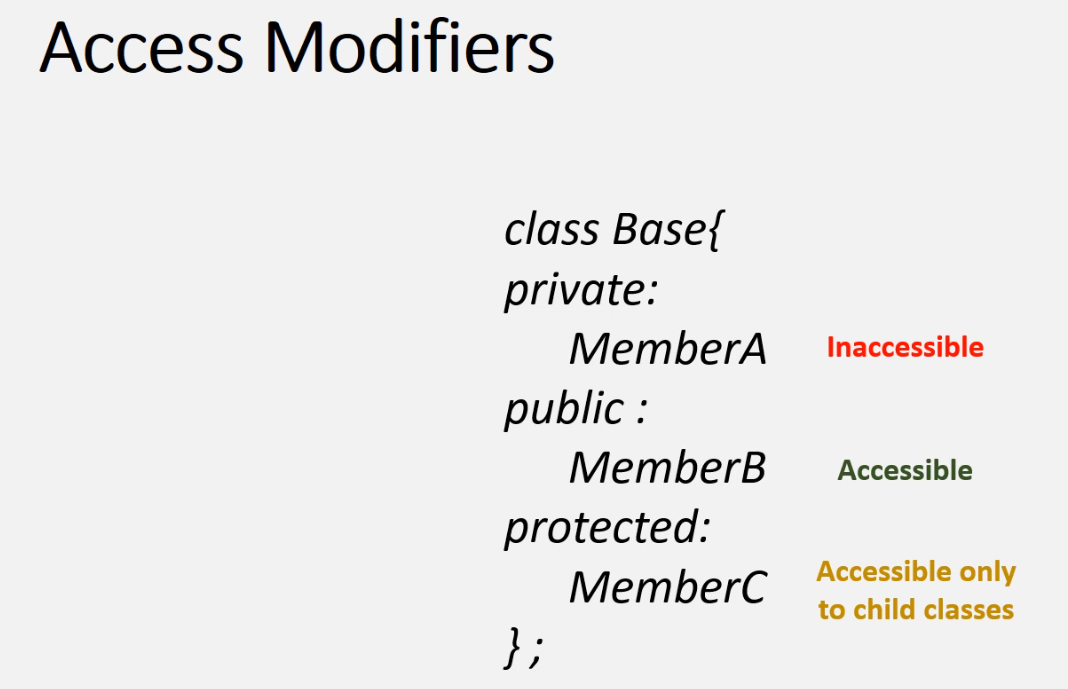
TIP
Protected members is accessible only to the class and its child classes. This means it is accessible only in the hierarchy and not outside it.
Public inheritance:
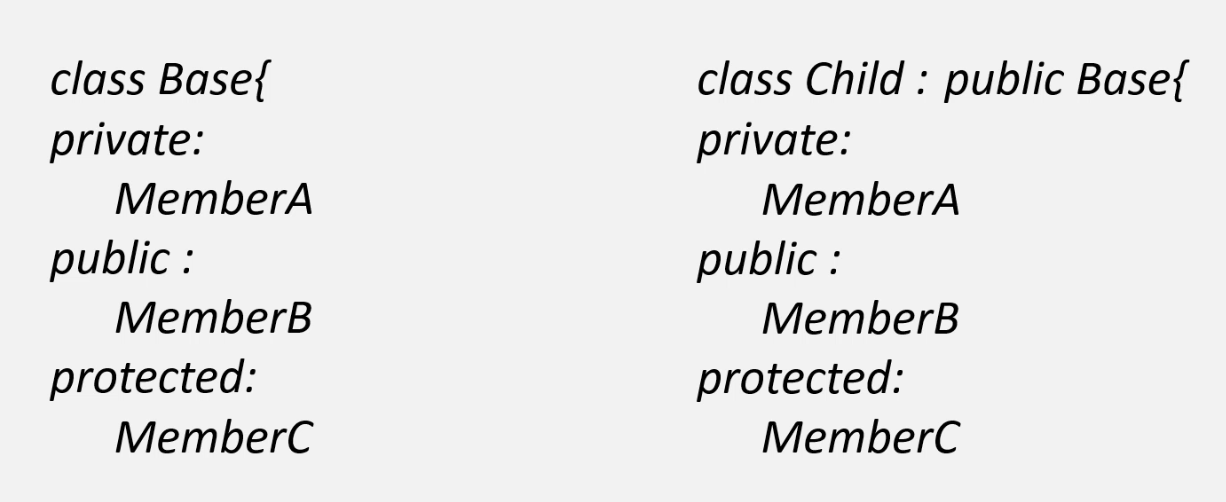
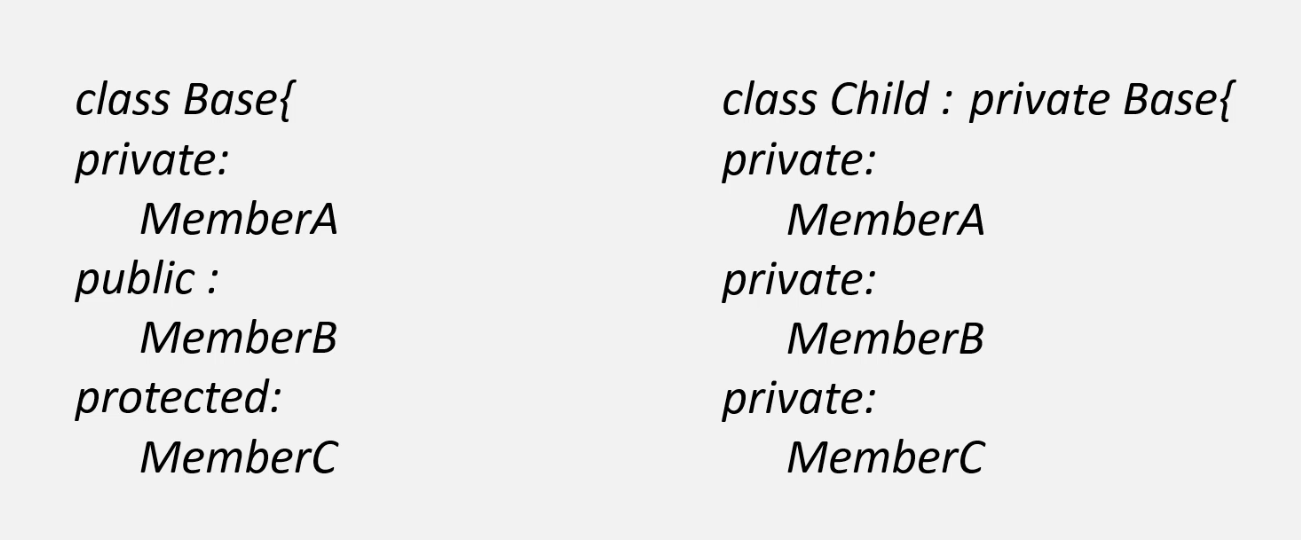
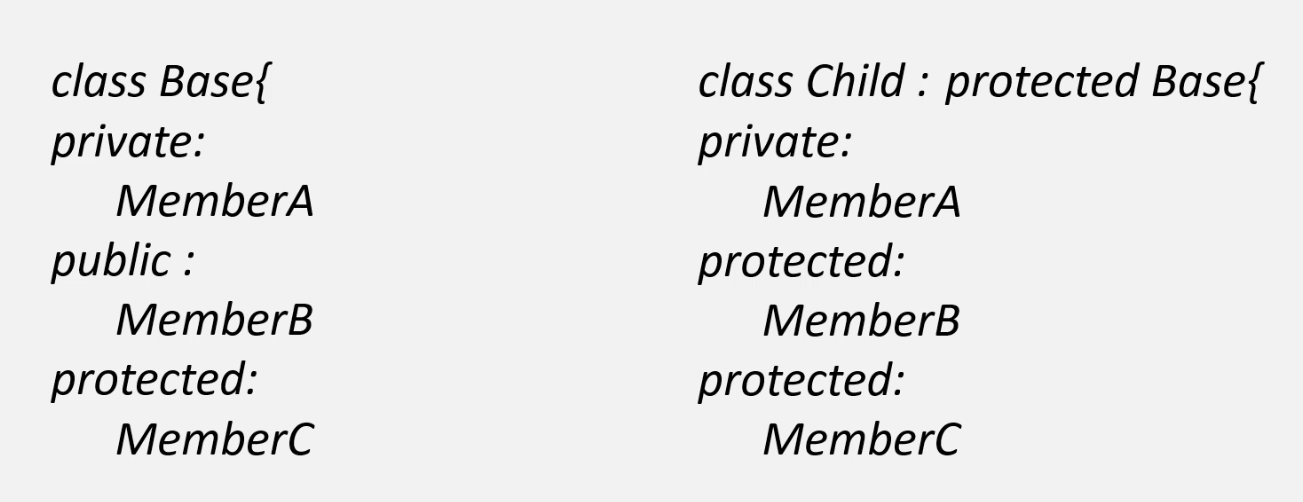
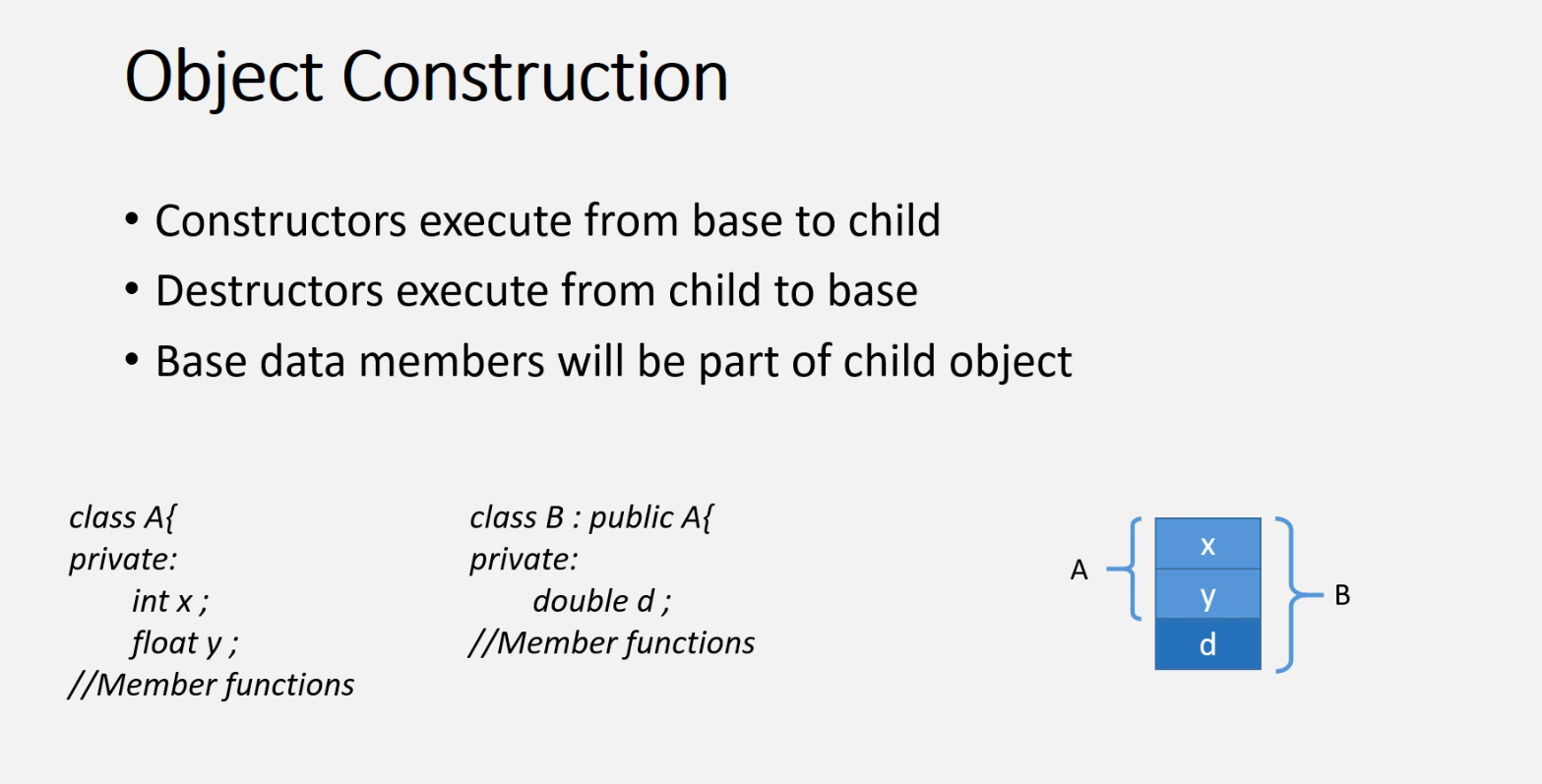