# Design Patterns
# Creational Design Patterns
# Singleton
Ensure a class only has one instance, and provide a global point of access to it.
# Implementation
- The class is made responsible for its own instance
- It intercepts the call for construction and returns a single instance
- Same instance is returned every time
- Therefore, direct construction of objects is disabled
- The class creates its own instance which is provided to the clients
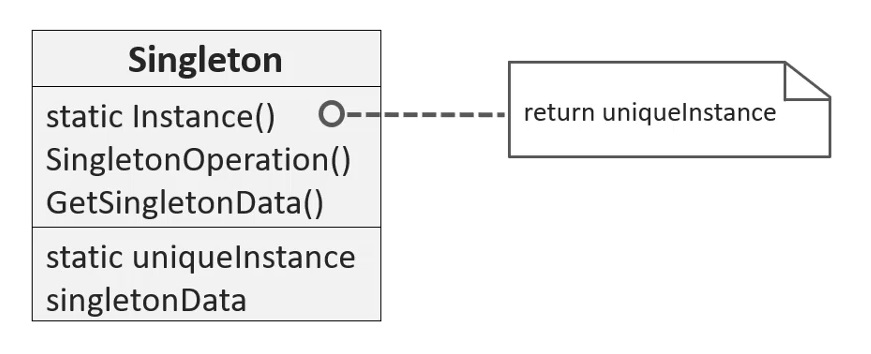
Simple Implementation
velocity.h
#ifndef VELOCITY_H
#define VELOCITY_H
class Velocity
{
Velocity() = default;
static Velocity m_Instance;
public:
static Velocity & Instance();
void MethodA();
void MethodB();
};
#endif // VELOCITY_H
1
2
3
4
5
6
7
8
9
10
11
12
13
14
2
3
4
5
6
7
8
9
10
11
12
13
14
velocity.cpp
#include "velocity.h"
Velocity Velocity::m_Instance;
Velocity& Velocity::Instance()
{
return m_Instance;
}
void Velocity::MethodA()
{
}
void Velocity::MethodB()
{
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18