# C++ Best Practices
C++ Best Practices for Developers
# New Features
C++11:
- Lambdas
- Auto type deduction
- List initialization
- Smart Pointers
- Move semantics
C++14:
- Type deduction
- Binary Literals
- Generic Lambda expressions
C++17:
- Structured binding
- Nested namespaces
- Inline Variables
- Constexpr lambda
# Compiler warnings
its good to take a look at the compiler's website to know more about the warnings, here are some recommended ones:
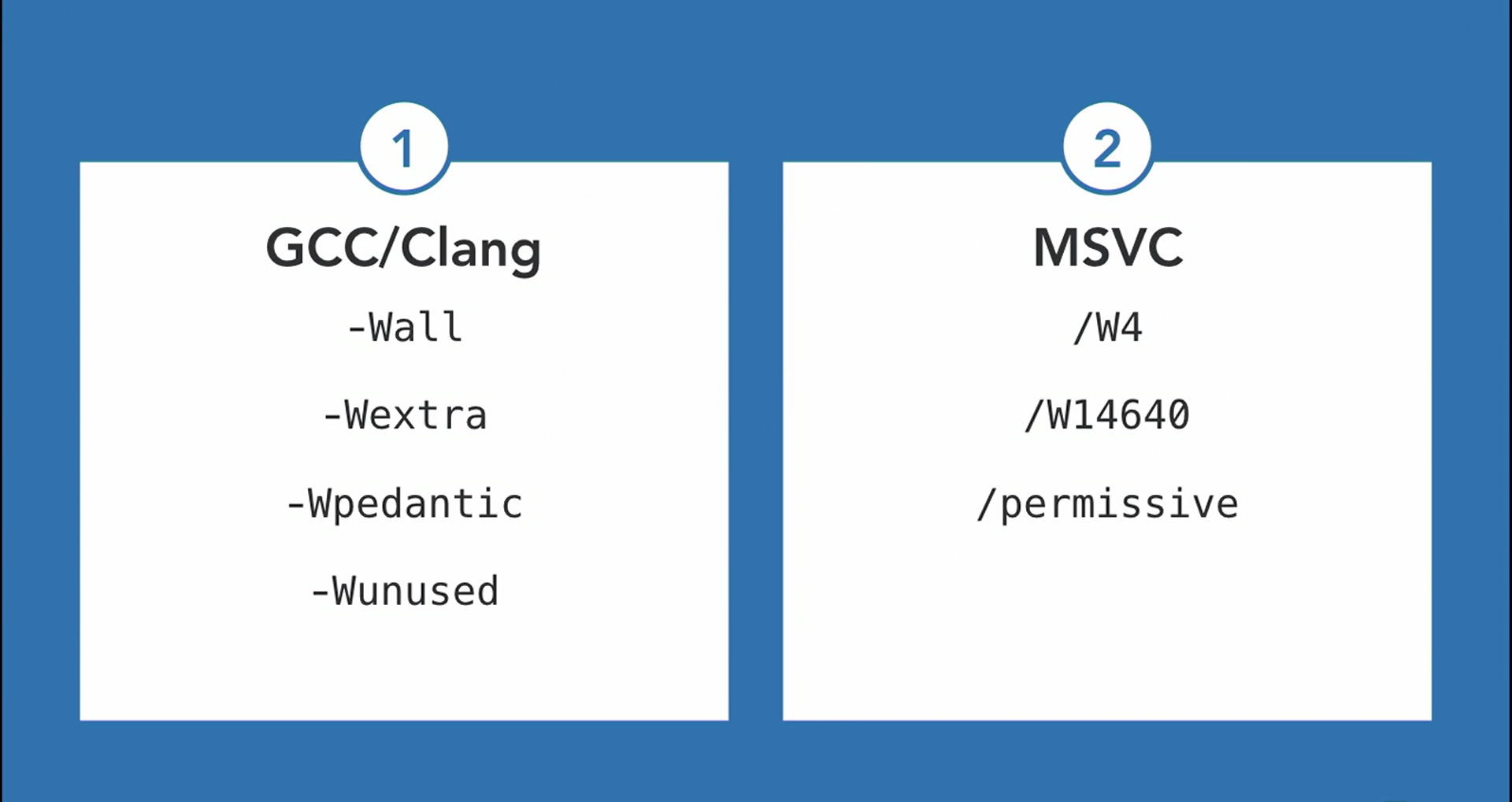
Static Analyzers also helps, here are some, but there are dozens more available.
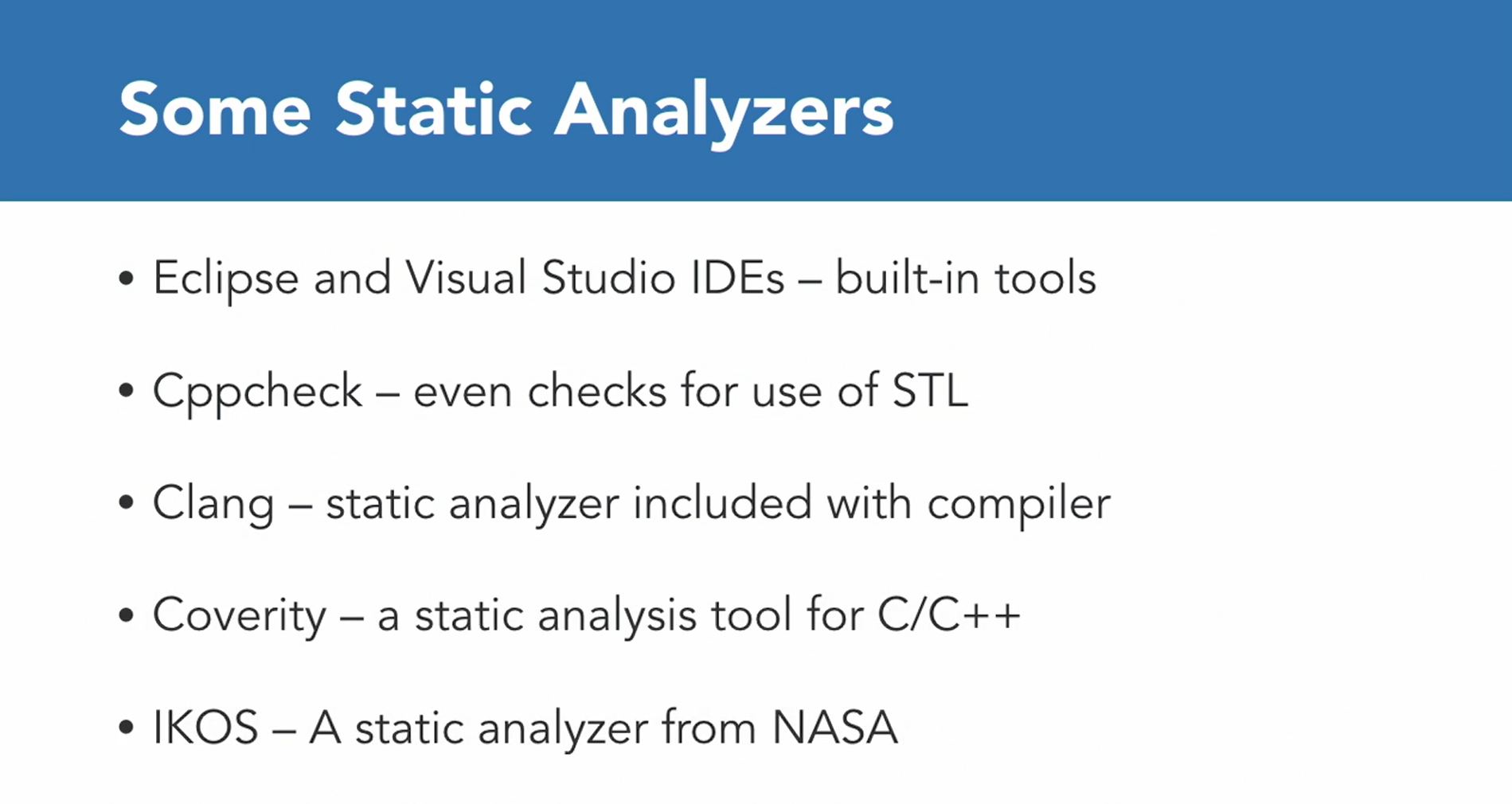
# Cppcheck
http://cppcheck.sourceforge.net
It runs from the command line.
sudo apt-get install cppcheck
Open the folder with the code on the command line and execute:
cppcheck --enable=all ./*.cpp
Watch for the raw loop:
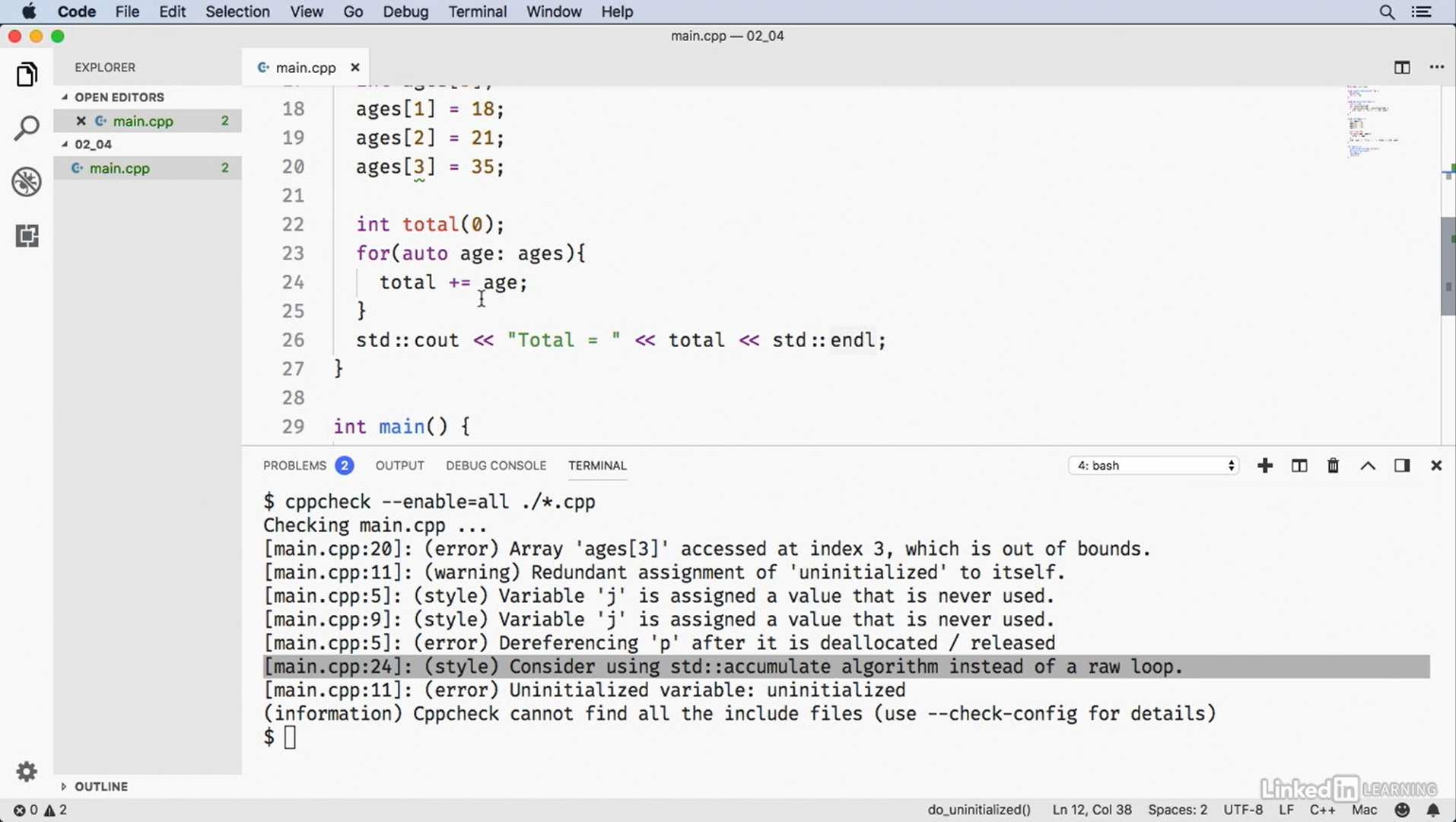
# Compiling the code
We can from the command line execute:
clang++ -std=c++17 -Wall main.cpp
# Using auto
auto tells the compiler to deduce the type of a variable
auto forces initialization of variables
if you need a specific type use auto with type initialization
Don't use auto to hide information
Example:
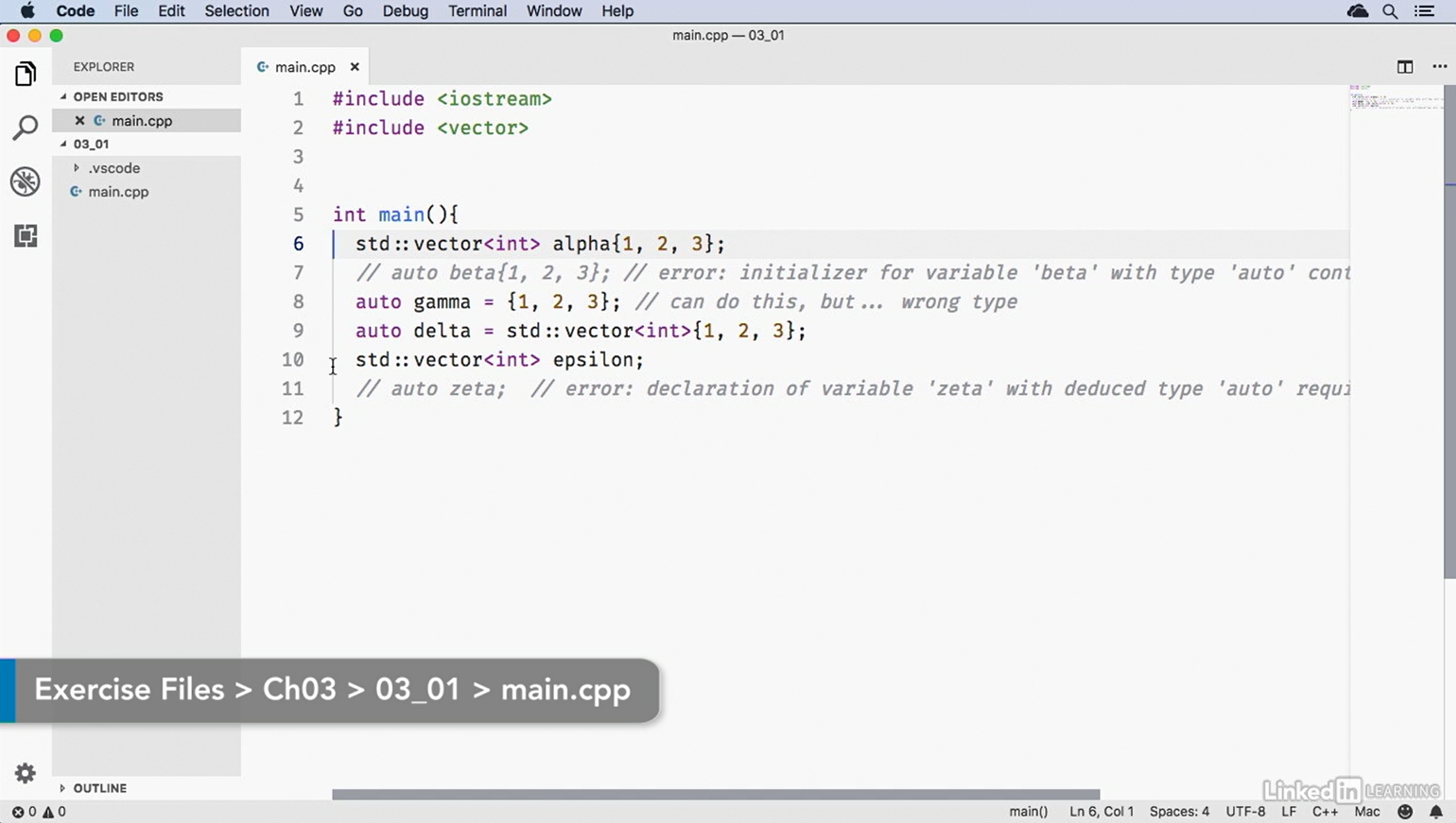
The right way will be using auto and defining the specific type, in this case a vector of int.
auto delta = std::vector<int>{1, 2, 3};
auto without initialization is always an error.
# Range based "for" loops
C++ can iterate over a range of values automatically, no need for indexing. Like STL containers, strings and arrays.
You can add support of range-based for loops to your own types by supporting the iterator interface.
Prefer range based for loops over traditional for loops
#include <iostream>
#include <vector>
#include <map>
int main() {
std::vector<int> v{0, 1, 2, 3, 4, 5};
for (auto i : v) {
std::cout << i << ' ';
}
std::cout << std::endl;
}
2
3
4
5
6
7
8
9
10
11